Setup programming and inbuilt JTAG debugging for an ESP32-C3 on Platformio.
- This is a ‘your results may vary’ solution, due to unknown variation.
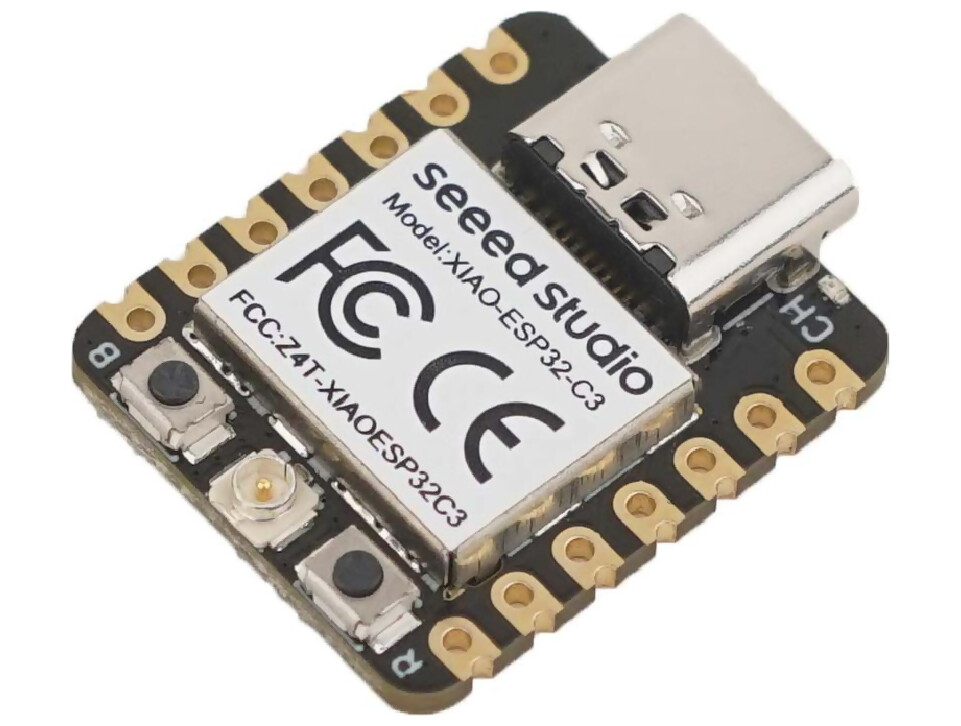
Connection
Check first that your ESP32-C3 is recognised when connected via USB (Linux).
user@machine:~$ lsusb
...
Bus 008 Device 033: ID 303a:1001 Espressif USB JTAG/serial debug unit
...
platformio.ini
Some build flags are required if you wish to enable serial output over USB, at the bitrate set by monitor_speed
.
The on-chip CMSIS-DAP device handles uploading for debugging, JTAG debugging, and can also flash code for non-debugger uploads if required.
For non-debugger builds, we can choose to use the USB CDC Serial firmware upload tool, which affords us some extra project statistics.
; PlatformIO Project Configuration File
;
; Build options: build flags, source filter
; Upload options: custom upload port, speed and extra flags
; Library options: dependencies, extra library storages
; Advanced options: extra scripting
;
; Please visit documentation for the other options and examples
; https://docs.platformio.org/page/projectconf.html
[env:esp32-c3-devkitm-1]
platform = espressif32
board = esp32-c3-devkitm-1
framework = arduino
monitor_speed=115200
build_flags =
-D ARDUINO_USB_MODE=1 ; Enable USB hardware (if used).
-D ARDUINO_USB_CDC_ON_BOOT=1 ; Enable CDC (Communications Device
; Class) functionality on boot,
; enabling USB serial communication.
debug_tool = cmsis-dap ; on-chip JTAG debug
; upload_protocol = esp-builtin ; on-chip JTAG upload (less feedback)
upload_protocol = esptool ; USB serial upload
debug_init_break = tbreak setup
debug_server =
$PLATFORMIO_CORE_DIR/packages/tool-openocd-esp32/bin/openocd
-f $PLATFORMIO_CORE_DIR/packages/tool-openocd-esp32/share/openocd/scripts/board/esp32c3-builtin.cfg
build and upload via USB serial
Remember that you can always enter program upload mode manually by RESETting the device with the BOOT switch depressed, in cases where previously programmed serial configuration prevents automatic CDC uploads.
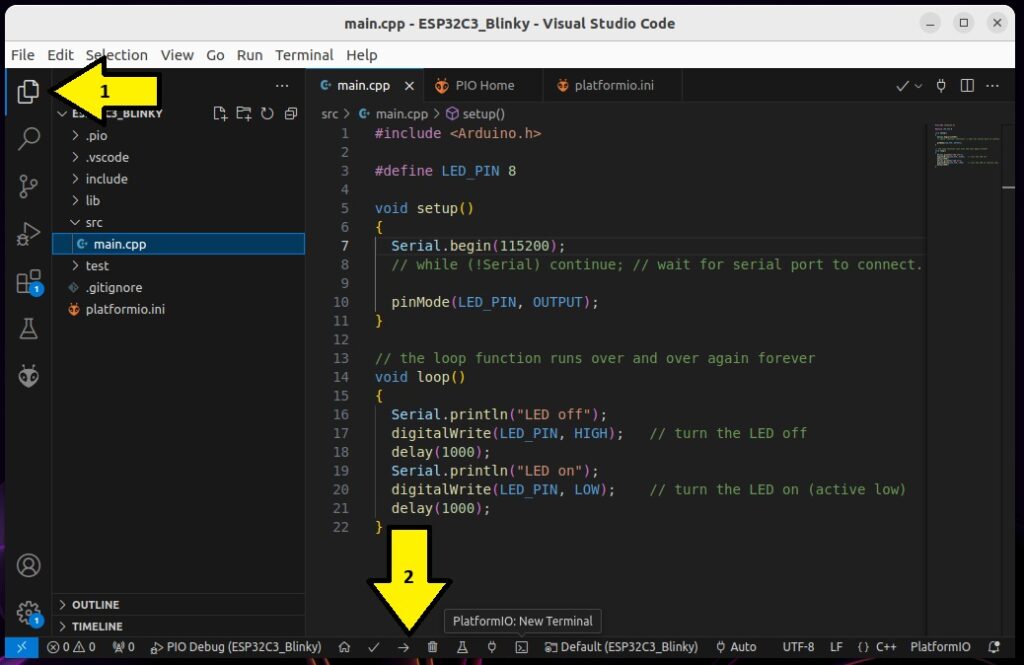
monitor serial
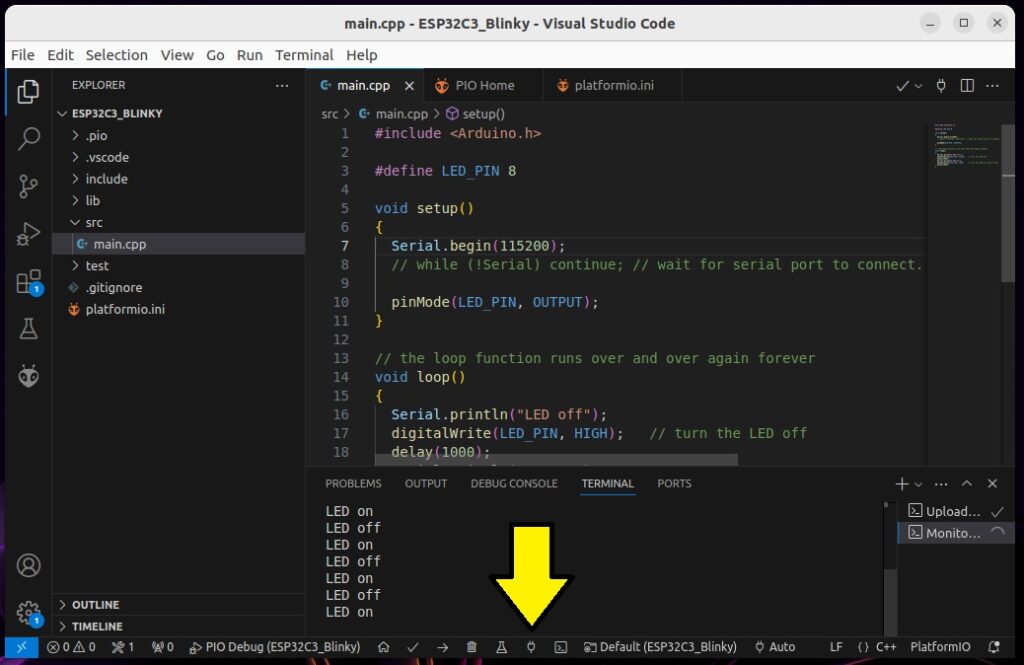
build for debugging then flash by JTAG
Full rebuild and upload produces lengthy wait, then blue ‘working’ indicator stops and the debugger control pallet appears.
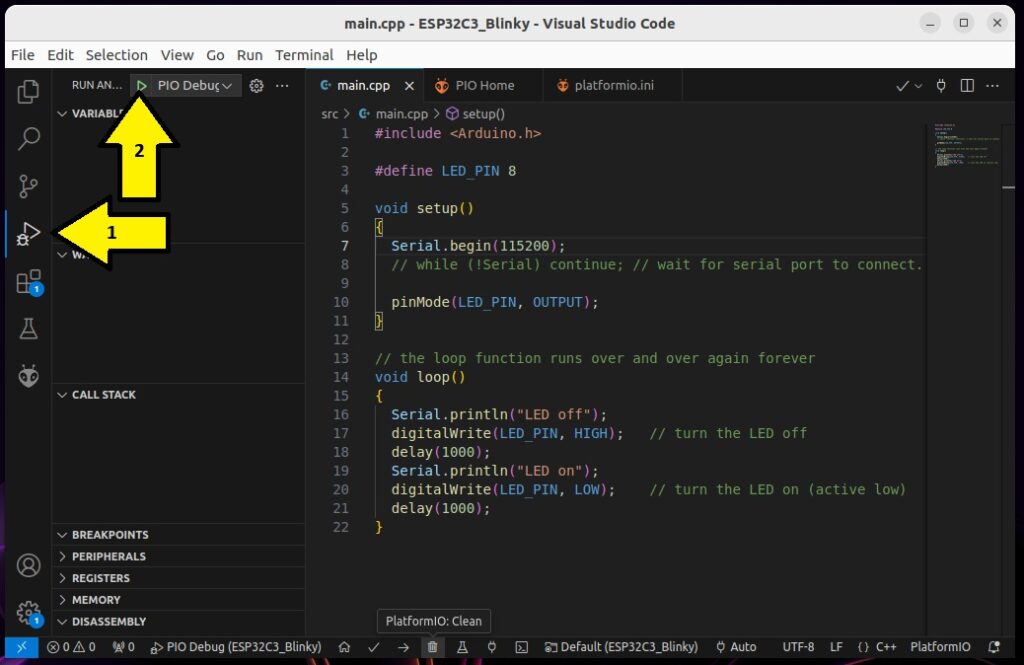
set breakpoint and debug via JTAG
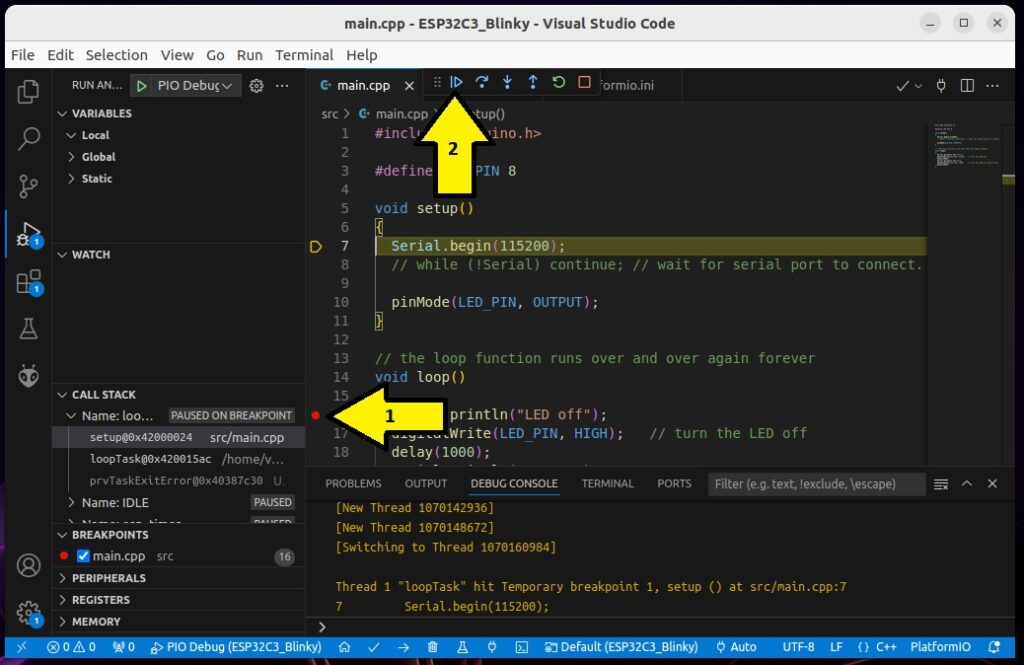
If you’ve found this compilation useful, then your assistance in helping others find it will be both benevolent and appreciated.
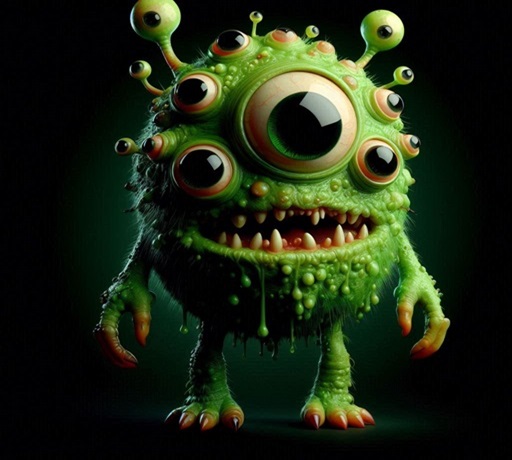